Use Case
C++ AWS S3 Client/Service. Use this client to perform S3 select operations on Parquet file stored on AWS S3. Integrates with the Boost Beast HTTP Service and Router and enabled S3 Select over HTTP(s).
Sample Usage of AWS S3 Select: Sravz YTD Page
Session 1:
- Perform S3 Select
- Perform S3 Select over HTTP
Libraries Used
Session 1
Dataflow Diagram
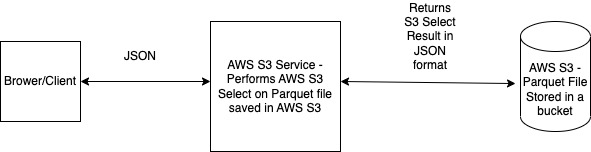