Use Case
Websocket (Session 1) -> Process Quotes (Session 2) -> Redis Timeseries (Session 3)
Use this programs to read stock quotes from WebSocket and store the timeseries in Redis Time Series Database
Libraries Used
- C++ Boost ASIO
- C++ Boost Beast
- hiredis
- Redis Plus Plus
Session 1
Code explanation video
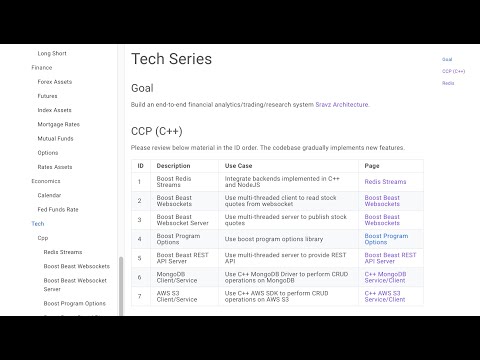
Source Code - Reads from WebSocket
Session 2
Code explanation video
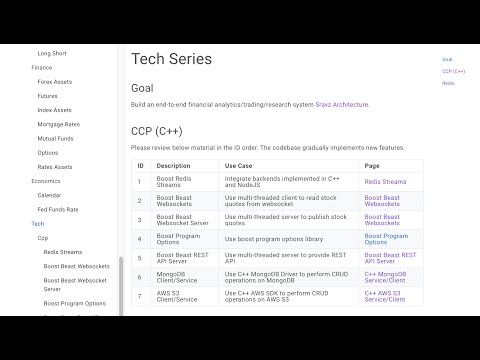
Source Code - Common Utils.h used across all files
Source Code - Reads from WebSocket and Publishes to Redis Topic
Source Code - Boost ASIO Threadpool - Redis Plus Plus Redis Subscriber
Session 3
Code explanation video
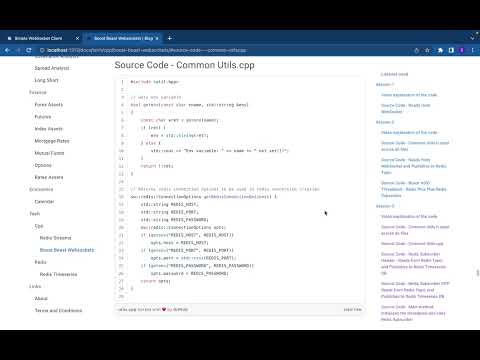
Source Code - Common Utils.h used across all files
Source Code - Common Utils.cpp
Source Code - Redis Subscriber Header - Reads from Redis Topic and Publishes to Redis Timeseries DB
Source Code - Redis Subscriber CPP- Reads from Redis Topic and Publishes to Redis Timeseries DB
Source Code - Main method, initializes the threadpool and calls Redis Subscriber
comments powered by