Use Case
Boost Beast based WebSocket Server
Use this programs to perform websocket calls.
Session 1:
- Updated boost beast multi chat example.
- Parses the HTTP request and sends a response text to the client.
Libraries Used
- C++ Boost ASIO
- C++ Boost Beast
- hiredis
- C++ JWT
- C++ Boost URL
References
Session 1
Thread Model
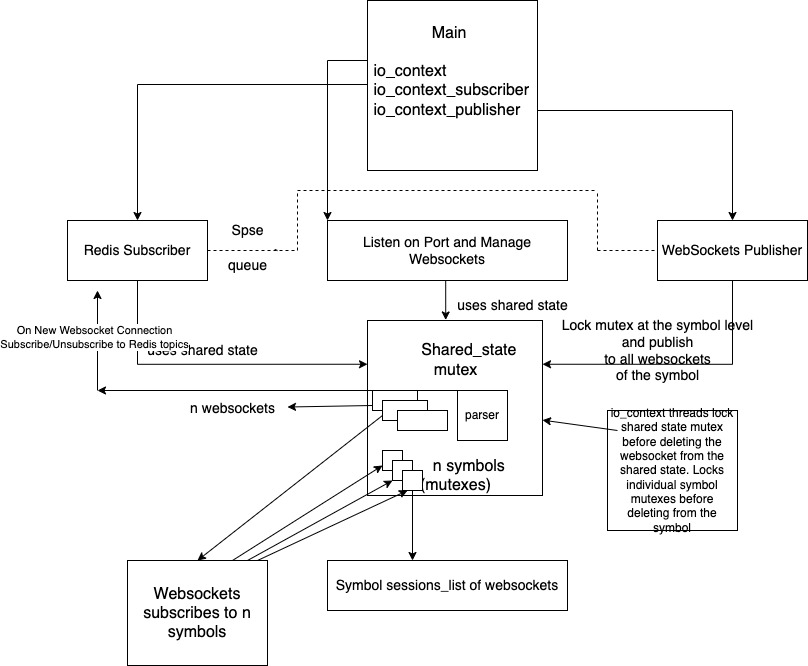